How to Call a Function Again if False Python
Spotter Now This tutorial has a related video course created by the Real Python squad. Watch it together with the written tutorial to deepen your understanding: How to Use Python Lambda Functions
Python and other languages like Java, C#, and fifty-fifty C++ have had lambda functions added to their syntax, whereas languages like LISP or the ML family of languages, Haskell, OCaml, and F#, utilize lambdas as a core concept.
Python lambdas are little, anonymous functions, subject to a more restrictive only more concise syntax than regular Python functions.
By the cease of this article, you lot'll know:
- How Python lambdas came to exist
- How lambdas compare with regular part objects
- How to write lambda functions
- Which functions in the Python standard library leverage lambdas
- When to use or avoid Python lambda functions
This tutorial is mainly for intermediate to experienced Python programmers, simply it is accessible to whatever curious minds with interest in programming and lambda calculus.
All the examples included in this tutorial have been tested with Python iii.7.
Lambda Calculus
Lambda expressions in Python and other programming languages have their roots in lambda calculus, a model of computation invented by Alonzo Church. You'll uncover when lambda calculus was introduced and why information technology's a fundamental concept that ended up in the Python ecosystem.
History
Alonzo Church formalized lambda calculus, a linguistic communication based on pure abstraction, in the 1930s. Lambda functions are also referred to as lambda abstractions, a directly reference to the abstraction model of Alonzo Church's original creation.
Lambda calculus tin encode any computation. Information technology is Turing consummate, but contrary to the concept of a Turing machine, it is pure and does not keep whatsoever land.
Functional languages go their origin in mathematical logic and lambda calculus, while imperative programming languages embrace the state-based model of ciphering invented by Alan Turing. The two models of computation, lambda calculus and Turing machines, can be translated into each another. This equivalence is known as the Church-Turing hypothesis.
Functional languages directly inherit the lambda calculus philosophy, adopting a declarative approach of programming that emphasizes abstraction, data transformation, composition, and purity (no state and no side effects). Examples of functional languages include Haskell, Lisp, or Erlang.
By contrast, the Turing Machine led to imperative programming institute in languages like Fortran, C, or Python.
The imperative mode consists of programming with statements, driving the menses of the programme step by step with detailed instructions. This approach promotes mutation and requires managing state.
The separation in both families presents some nuances, as some functional languages incorporate imperative features, like OCaml, while functional features take been permeating the imperative family of languages in particular with the introduction of lambda functions in Java, or Python.
Python is not inherently a functional linguistic communication, but information technology adopted some functional concepts early on. In January 1994, map()
, filter()
, reduce()
, and the lambda
operator were added to the language.
First Example
Here are a few examples to requite yous an appetite for some Python code, functional fashion.
The identity office, a function that returns its statement, is expressed with a standard Python role definition using the keyword def
equally follows:
>>>
>>> def identity ( x ): ... render x
identity()
takes an argument x
and returns it upon invocation.
In contrast, if you use a Python lambda structure, you lot go the following:
In the case above, the expression is composed of:
- The keyword:
lambda
- A leap variable:
x
- A torso:
ten
You lot can write a slightly more elaborated instance, a function that adds i
to an argument, as follows:
You can apply the office above to an argument by surrounding the function and its argument with parentheses:
>>>
>>> ( lambda ten : x + 1 )( 2 ) 3
Reduction is a lambda calculus strategy to compute the value of the expression. In the current case, it consists of replacing the bound variable ten
with the argument 2
:
(lambda x: x + 1)(2) = lambda ii: 2 + 1 = 2 + 1 = iii
Considering a lambda part is an expression, it can exist named. Therefore y'all could write the previous code equally follows:
>>>
>>> add_one = lambda x : x + 1 >>> add_one ( 2 ) 3
The above lambda function is equivalent to writing this:
def add_one ( ten ): return 10 + i
These functions all take a single argument. You may have noticed that, in the definition of the lambdas, the arguments don't have parentheses around them. Multi-argument functions (functions that take more than one argument) are expressed in Python lambdas by listing arguments and separating them with a comma (,
) but without surrounding them with parentheses:
>>>
>>> full_name = lambda starting time , last : f 'Full proper noun: { first . championship () } { last . title () } ' >>> full_name ( 'guido' , 'van rossum' ) 'Total name: Guido Van Rossum'
The lambda function assigned to full_name
takes 2 arguments and returns a cord interpolating the two parameters first
and final
. Every bit expected, the definition of the lambda lists the arguments with no parentheses, whereas calling the part is done exactly like a normal Python function, with parentheses surrounding the arguments.
Anonymous Functions
The following terms may be used interchangeably depending on the programming language type and culture:
- Anonymous functions
- Lambda functions
- Lambda expressions
- Lambda abstractions
- Lambda course
- Function literals
For the rest of this commodity after this department, you lot'll mostly meet the term lambda function.
Taken literally, an anonymous function is a role without a name. In Python, an anonymous part is created with the lambda
keyword. More loosely, it may or non exist assigned a proper name. Consider a two-argument anonymous function defined with lambda
merely not bound to a variable. The lambda is non given a proper noun:
>>>
>>> lambda x , y : x + y
The office in a higher place defines a lambda expression that takes two arguments and returns their sum.
Other than providing you with the feedback that Python is perfectly fine with this form, it doesn't lead to any practical apply. You could invoke the function in the Python interpreter:
The example to a higher place is taking advantage of the interactive interpreter-only feature provided via the underscore (_
). Encounter the note below for more details.
You could non write similar code in a Python module. Consider the _
in the interpreter equally a side effect that you took advantage of. In a Python module, y'all would assign a proper noun to the lambda, or you would pass the lambda to a function. You'll utilize those two approaches later in this commodity.
Another pattern used in other languages like JavaScript is to immediately execute a Python lambda function. This is known as an Immediately Invoked Role Expression (IIFE, pronounce "iffy"). Hither's an example:
>>>
>>> ( lambda ten , y : x + y )( 2 , 3 ) 5
The lambda function to a higher place is divers and so immediately called with 2 arguments (two
and 3
). Information technology returns the value 5
, which is the sum of the arguments.
Several examples in this tutorial utilize this format to highlight the anonymous aspect of a lambda function and avert focusing on lambda
in Python every bit a shorter mode of defining a function.
Python does not encourage using immediately invoked lambda expressions. It but results from a lambda expression beingness callable, unlike the body of a normal function.
Lambda functions are often used with higher-social club functions, which take one or more functions as arguments or return one or more functions.
A lambda function can be a higher-order function by taking a function (normal or lambda) every bit an statement like in the following contrived example:
>>>
>>> high_ord_func = lambda x , func : x + func ( x ) >>> high_ord_func ( ii , lambda ten : x * x ) half dozen >>> high_ord_func ( 2 , lambda x : x + iii ) 7
Python exposes higher-order functions equally born functions or in the standard library. Examples include map()
, filter()
, functools.reduce()
, as well as key functions like sort()
, sorted()
, min()
, and max()
. You lot'll use lambda functions together with Python college-order functions in Appropriate Uses of Lambda Expressions.
Python Lambda and Regular Functions
This quote from the Python Blueprint and History FAQ seems to set the tone most the overall expectation regarding the usage of lambda functions in Python:
Dissimilar lambda forms in other languages, where they add functionality, Python lambdas are only a shorthand annotation if y'all're also lazy to define a function. (Source)
Nevertheless, don't permit this statement deter you from using Python's lambda
. At first glance, you may accept that a lambda function is a part with some syntactic sugar shortening the code to define or invoke a function. The following sections highlight the commonalities and subtle differences between normal Python functions and lambda functions.
Functions
At this betoken, you may wonder what fundamentally distinguishes a lambda part spring to a variable from a regular function with a single render
line: under the surface, almost zippo. Let's verify how Python sees a function built with a single render statement versus a office constructed every bit an expression (lambda
).
The dis
module exposes functions to analyze Python bytecode generated by the Python compiler:
>>>
>>> import dis >>> add = lambda x , y : ten + y >>> blazon ( add ) <class 'role'> >>> dis . dis ( add ) i 0 LOAD_FAST 0 (x) ii LOAD_FAST one (y) 4 BINARY_ADD half-dozen RETURN_VALUE >>> add <role <lambda> at 0x7f30c6ce9ea0>
You can see that dis()
expose a readable version of the Python bytecode assuasive the inspection of the low-level instructions that the Python interpreter volition utilise while executing the program.
Now meet it with a regular function object:
>>>
>>> import dis >>> def add ( x , y ): return x + y >>> type ( add together ) <grade 'function'> >>> dis . dis ( add together ) 1 0 LOAD_FAST 0 (x) 2 LOAD_FAST 1 (y) 4 BINARY_ADD half dozen RETURN_VALUE >>> add <part add together at 0x7f30c6ce9f28>
The bytecode interpreted by Python is the same for both functions. But you may observe that the naming is different: the part name is add
for a part defined with def
, whereas the Python lambda office is seen as lambda
.
Traceback
You lot saw in the previous department that, in the context of the lambda function, Python did not provide the name of the function, but only <lambda>
. This tin exist a limitation to consider when an exception occurs, and a traceback shows only <lambda>
:
>>>
>>> div_zero = lambda x : 10 / 0 >>> div_zero ( 2 ) Traceback (most recent call last): File "<stdin>" , line i , in < module > File "<stdin>" , line 1 , in < lambda > ZeroDivisionError: division past zero
The traceback of an exception raised while a lambda function is executed only identifies the function causing the exception as <lambda>
.
Hither'south the aforementioned exception raised by a normal function:
>>>
>>> def div_zero ( x ): return x / 0 >>> div_zero ( ii ) Traceback (most recent call concluding): File "<stdin>" , line 1 , in < module > File "<stdin>" , line 1 , in div_zero ZeroDivisionError: division by zero
The normal function causes a like mistake but results in a more precise traceback because it gives the role proper name, div_zero
.
Syntax
Every bit you saw in the previous sections, a lambda form presents syntactic distinctions from a normal function. In particular, a lambda function has the following characteristics:
- Information technology can only contain expressions and can't include statements in its body.
- It is written as a single line of execution.
- It does non back up type annotations.
- It can be immediately invoked (IIFE).
No Statements
A lambda function tin can't contain any statements. In a lambda role, statements like return
, pass
, assert
, or heighten
will raise a SyntaxError
exception. Hither's an instance of adding assert
to the trunk of a lambda:
>>>
>>> ( lambda x : assert x == two )( 2 ) File "<input>", line ane ( lambda 10 : assert x == 2 )( two ) ^ SyntaxError: invalid syntax
This contrived example intended to affirm
that parameter ten
had a value of 2
. Simply, the interpreter identifies a SyntaxError
while parsing the code that involves the statement assert
in the body of the lambda
.
Unmarried Expression
In contrast to a normal function, a Python lambda function is a single expression. Although, in the torso of a lambda
, you can spread the expression over several lines using parentheses or a multiline string, it remains a single expression:
>>>
>>> ( lambda 10 : ... ( 10 % ii and 'odd' or 'even' ))( iii ) 'odd'
The example above returns the string 'odd'
when the lambda argument is odd, and 'fifty-fifty'
when the argument is fifty-fifty. Information technology spreads across ii lines because information technology is contained in a set of parentheses, but it remains a single expression.
Type Annotations
If you've started adopting blazon hinting, which is now available in Python, then you accept some other good reason to adopt normal functions over Python lambda functions. Check out Python Blazon Checking (Guide) to become learn more virtually Python blazon hints and type checking. In a lambda function, there is no equivalent for the following:
def full_name ( start : str , last : str ) -> str : return f ' { first . championship () } { last . championship () } '
Any type mistake with full_name()
can be caught by tools like mypy
or pyre
, whereas a SyntaxError
with the equivalent lambda function is raised at runtime:
>>>
>>> lambda first : str , terminal : str : first . title () + " " + concluding . championship () -> str File "<stdin>", line 1 lambda starting time : str , last : str : first . championship () + " " + last . title () -> str SyntaxError: invalid syntax
Like trying to include a statement in a lambda, calculation type annotation immediately results in a SyntaxError
at runtime.
IIFE
Yous've already seen several examples of immediately invoked part execution:
>>>
>>> ( lambda ten : 10 * x )( 3 ) nine
Outside of the Python interpreter, this feature is probably non used in do. Information technology'due south a direct consequence of a lambda function being callable equally it is divers. For example, this allows you to pass the definition of a Python lambda expression to a higher-lodge office like map()
, filter()
, or functools.reduce()
, or to a key function.
Arguments
Like a normal role object defined with def
, Python lambda expressions support all the different means of passing arguments. This includes:
- Positional arguments
- Named arguments (sometimes called keyword arguments)
- Variable listing of arguments (often referred to equally varargs)
- Variable list of keyword arguments
- Keyword-merely arguments
The following examples illustrate options open to you in order to pass arguments to lambda expressions:
>>>
>>> ( lambda x , y , z : 10 + y + z )( ane , 2 , 3 ) half-dozen >>> ( lambda x , y , z = 3 : 10 + y + z )( 1 , two ) 6 >>> ( lambda x , y , z = 3 : 10 + y + z )( 1 , y = ii ) half-dozen >>> ( lambda * args : sum ( args ))( 1 , 2 , 3 ) half-dozen >>> ( lambda ** kwargs : sum ( kwargs . values ()))( one = ane , two = ii , three = 3 ) 6 >>> ( lambda x , * , y = 0 , z = 0 : x + y + z )( ane , y = 2 , z = 3 ) 6
Decorators
In Python, a decorator is the implementation of a pattern that allows adding a behavior to a part or a class. It is usually expressed with the @decorator
syntax prefixing a part. Here's a contrived instance:
def some_decorator ( f ): def wraps ( * args ): print ( f "Calling function ' { f . __name__ } '" ) return f ( args ) return wraps @some_decorator def decorated_function ( x ): print ( f "With argument ' { x } '" )
In the instance above, some_decorator()
is a function that adds a behavior to decorated_function()
, so that invoking decorated_function("Python")
results in the post-obit output:
Calling office 'decorated_function' With argument 'Python'
decorated_function()
only prints With argument 'Python'
, but the decorator adds an actress behavior that also prints Calling role 'decorated_function'
.
A decorator can be applied to a lambda. Although it'southward not possible to decorate a lambda with the @decorator
syntax, a decorator is just a function, then it tin call the lambda function:
1 # Defining a decorator 2 def trace ( f ): 3 def wrap ( * args , ** kwargs ): 4 impress ( f "[TRACE] func: { f . __name__ } , args: { args } , kwargs: { kwargs } " ) 5 return f ( * args , ** kwargs ) 6 vii return wrap eight ix # Applying decorator to a function 10 @trace xi def add_two ( x ): 12 return x + two xiii 14 # Calling the busy function 15 add_two ( three ) 16 17 # Applying decorator to a lambda 18 print (( trace ( lambda x : x ** two ))( 3 ))
add_two()
, decorated with @trace
on line 11, is invoked with argument three
on line fifteen. By contrast, on line xviii, a lambda role is immediately involved and embedded in a telephone call to trace()
, the decorator. When you lot execute the code higher up you obtain the following:
[TRACE] func: add_two, args: (three,), kwargs: {} [TRACE] func: <lambda>, args: (iii,), kwargs: {} 9
Meet how, as y'all've already seen, the name of the lambda office appears as <lambda>
, whereas add_two
is clearly identified for the normal function.
Decorating the lambda function this way could be useful for debugging purposes, possibly to debug the behavior of a lambda function used in the context of a higher-order function or a key role. Let'due south see an example with map()
:
list ( map ( trace ( lambda 10 : x * ii ), range ( 3 )))
The kickoff argument of map()
is a lambda that multiplies its argument past 2
. This lambda is decorated with trace()
. When executed, the example above outputs the post-obit:
[TRACE] Calling <lambda> with args (0,) and kwargs {} [TRACE] Calling <lambda> with args (ane,) and kwargs {} [TRACE] Calling <lambda> with args (2,) and kwargs {} [0, two, iv]
The upshot [0, 2, 4]
is a list obtained from multiplying each element of range(iii)
. For now, consider range(3)
equivalent to the list [0, 1, 2]
.
You will be exposed to map()
in more than details in Map.
A lambda tin can also be a decorator, but information technology'southward non recommended. If you detect yourself needing to do this, consult PEP viii, Programming Recommendations.
For more on Python decorators, bank check out Primer on Python Decorators.
Closure
A closure is a role where every free variable, everything except parameters, used in that function is bound to a specific value divers in the enclosing scope of that role. In effect, closures define the surround in which they run, and so can be called from anywhere.
The concepts of lambdas and closures are non necessarily related, although lambda functions can be closures in the aforementioned mode that normal functions can also be closures. Some languages take special constructs for closure or lambda (for instance, Groovy with an anonymous block of code as Closure object), or a lambda expression (for example, Java Lambda expression with a limited option for closure).
Hither'south a closure constructed with a normal Python function:
ane def outer_func ( x ): 2 y = 4 3 def inner_func ( z ): 4 impress ( f "ten = { x } , y = { y } , z = { z } " ) five render x + y + z half dozen return inner_func 7 8 for i in range ( 3 ): ix closure = outer_func ( i ) ten print ( f "closure( { i + 5 } ) = { closure ( i + five ) } " )
outer_func()
returns inner_func()
, a nested function that computes the sum of iii arguments:
-
x
is passed as an argument toouter_func()
. -
y
is a variable local toouter_func()
. -
z
is an argument passed toinner_func()
.
To test the behavior of outer_func()
and inner_func()
, outer_func()
is invoked iii times in a for
loop that prints the following:
x = 0, y = iv, z = 5 closure(v) = 9 x = 1, y = 4, z = vi closure(vi) = xi x = ii, y = 4, z = 7 closure(seven) = 13
On line 9 of the code, inner_func()
returned by the invocation of outer_func()
is bound to the name closure
. On line 5, inner_func()
captures 10
and y
considering it has access to its embedding environment, such that upon invocation of the closure, it is able to operate on the two free variables x
and y
.
Similarly, a lambda
tin likewise exist a closure. Here'southward the same example with a Python lambda function:
1 def outer_func ( x ): 2 y = four 3 return lambda z : ten + y + z 4 five for i in range ( 3 ): 6 closure = outer_func ( i ) 7 print ( f "closure( { i + 5 } ) = { closure ( i + 5 ) } " )
When you execute the code in a higher place, you obtain the post-obit output:
closure(5) = 9 closure(six) = eleven closure(7) = xiii
On line 6, outer_func()
returns a lambda and assigns it to to the variable closure
. On line 3, the torso of the lambda role references 10
and y
. The variable y
is available at definition fourth dimension, whereas x
is defined at runtime when outer_func()
is invoked.
In this situation, both the normal function and the lambda comport similarly. In the side by side section, you'll see a situation where the beliefs of a lambda tin can be deceptive due to its evaluation time (definition fourth dimension vs runtime).
Evaluation Time
In some situations involving loops, the behavior of a Python lambda function as a closure may be counterintuitive. It requires understanding when free variables are bound in the context of a lambda. The post-obit examples demonstrate the departure when using a regular role vs using a Python lambda.
Examination the scenario first using a regular function:
>>>
1 >>> def wrap ( n ): 2 ... def f (): 3 ... print ( north ) iv ... return f 5 ... 6 >>> numbers = 'one' , 'two' , 'three' 7 >>> funcs = [] viii >>> for northward in numbers : 9 ... funcs . append ( wrap ( northward )) 10 ... xi >>> for f in funcs : 12 ... f () thirteen ... 14 i 15 two 16 three
In a normal function, n
is evaluated at definition fourth dimension, on line 9, when the function is added to the listing: funcs.append(wrap(n))
.
Now, with the implementation of the same logic with a lambda part, observe the unexpected behavior:
>>>
i >>> numbers = 'one' , '2' , 'three' 2 >>> funcs = [] iii >>> for n in numbers : 4 ... funcs . append ( lambda : print ( n )) 5 ... 6 >>> for f in funcs : seven ... f () viii ... nine three ten three eleven three
The unexpected event occurs because the free variable n
, as implemented, is jump at the execution time of the lambda expression. The Python lambda function on line 4 is a closure that captures north
, a free variable leap at runtime. At runtime, while invoking the office f
on line 7, the value of n
is three
.
To overcome this issue, y'all can assign the free variable at definition time as follows:
>>>
i >>> numbers = '1' , 'two' , 'three' 2 >>> funcs = [] 3 >>> for north in numbers : 4 ... funcs . suspend ( lambda north = due north : impress ( n )) five ... 6 >>> for f in funcs : 7 ... f () 8 ... 9 one 10 2 11 three
A Python lambda function behaves like a normal office in regard to arguments. Therefore, a lambda parameter can exist initialized with a default value: the parameter n
takes the outer n
as a default value. The Python lambda function could have been written as lambda x=n: impress(x)
and have the aforementioned result.
The Python lambda part is invoked without whatever argument on line 7, and it uses the default value due north
set at definition time.
Testing Lambdas
Python lambdas can exist tested similarly to regular functions. It's possible to use both unittest
and doctest
.
unittest
The unittest
module handles Python lambda functions similarly to regular functions:
import unittest addtwo = lambda ten : x + ii class LambdaTest ( unittest . TestCase ): def test_add_two ( cocky ): self . assertEqual ( addtwo ( 2 ), four ) def test_add_two_point_two ( self ): self . assertEqual ( addtwo ( 2.two ), 4.2 ) def test_add_three ( self ): # Should fail cocky . assertEqual ( addtwo ( three ), 6 ) if __name__ == '__main__' : unittest . primary ( verbosity = two )
LambdaTest
defines a test instance with 3 test methods, each of them exercising a exam scenario for addtwo()
implemented as a lambda function. The execution of the Python file lambda_unittest.py
that contains LambdaTest
produces the following:
$ python lambda_unittest.py test_add_three (__main__.LambdaTest) ... Fail test_add_two (__main__.LambdaTest) ... ok test_add_two_point_two (__main__.LambdaTest) ... ok ====================================================================== Neglect: test_add_three (__main__.LambdaTest) ---------------------------------------------------------------------- Traceback (most recent call final): File "lambda_unittest.py", line 18, in test_add_three cocky.assertEqual(addtwo(3), 6) AssertionError: 5 != 6 ---------------------------------------------------------------------- Ran 3 tests in 0.001s FAILED (failures=1)
As expected, nosotros have ii successful test cases and one failure for test_add_three
: the issue is v
, but the expected outcome was vi
. This failure is due to an intentional fault in the examination case. Changing the expected result from 6
to v
will satisfy all the tests for LambdaTest
.
doctest
The doctest
module extracts interactive Python code from docstring
to execute tests. Although the syntax of Python lambda functions does not support a typical docstring
, it is possible to assign a cord to the __doc__
chemical element of a named lambda:
addtwo = lambda 10 : x + 2 addtwo . __doc__ = """Add 2 to a number. >>> addtwo(2) iv >>> addtwo(2.2) 4.2 >>> addtwo(3) # Should fail 6 """ if __name__ == '__main__' : import doctest doctest . testmod ( verbose = Truthful )
The doctest
in the physician comment of lambda addtwo()
describes the same test cases as in the previous department.
When yous execute the tests via doctest.testmod()
, you go the following:
$ python lambda_doctest.py Trying: addtwo(2) Expecting: 4 ok Trying: addtwo(ii.2) Expecting: 4.2 ok Trying: addtwo(3) # Should fail Expecting: half dozen ********************************************************************** File "lambda_doctest.py", line 16, in __main__.addtwo Failed example: addtwo(iii) # Should fail Expected: half-dozen Got: v 1 items had no tests: __main__ ********************************************************************** 1 items had failures: 1 of iii in __main__.addtwo 3 tests in 2 items. 2 passed and i failed. ***Test Failed*** 1 failures.
The failed test results from the same failure explained in the execution of the unit tests in the previous section.
You can add a docstring
to a Python lambda via an assignment to __doc__
to document a lambda function. Although possible, the Python syntax ameliorate accommodates docstring
for normal functions than lambda functions.
For a comprehensive overview of unit of measurement testing in Python, you lot may desire to refer to Getting Started With Testing in Python.
Lambda Expression Abuses
Several examples in this commodity, if written in the context of professional Python lawmaking, would qualify as abuses.
If y'all observe yourself trying to overcome something that a lambda expression does not support, this is probably a sign that a normal function would be improve suited. The docstring
for a lambda expression in the previous section is a good example. Attempting to overcome the fact that a Python lambda function does not support statements is another red flag.
The next sections illustrate a few examples of lambda usages that should be avoided. Those examples might be situations where, in the context of Python lambda, the lawmaking exhibits the following blueprint:
- Information technology doesn't follow the Python style guide (PEP 8)
- It's cumbersome and difficult to read.
- Information technology's unnecessarily clever at the cost of difficult readability.
Raising an Exception
Trying to raise an exception in a Python lambda should make you recollect twice. There are some clever ways to practice and then, merely even something like the following is better to avoid:
>>>
>>> def throw ( ex ): raise ex >>> ( lambda : throw ( Exception ( 'Something bad happened' )))() Traceback (virtually recent call last): File "<stdin>" , line 1 , in < module > File "<stdin>" , line one , in < lambda > File "<stdin>" , line 1 , in throw Exception: Something bad happened
Because a statement is non syntactically right in a Python lambda body, the workaround in the example to a higher place consists of abstracting the statement phone call with a dedicated function throw()
. Using this type of workaround should exist avoided. If you encounter this blazon of code, you should consider refactoring the code to use a regular office.
Cryptic Style
As in any programming languages, you will notice Python code that tin exist difficult to read considering of the style used. Lambda functions, due to their conciseness, tin be conducive to writing code that is difficult to read.
The following lambda example contains several bad style choices:
>>>
>>> ( lambda _ : list ( map ( lambda _ : _ // 2 , _ )))([ 1 , ii , three , iv , 5 , 6 , seven , viii , 9 , 10 ]) [0, 1, 1, 2, 2, 3, three, 4, four, 5]
The underscore (_
) refers to a variable that you don't need to refer to explicitly. But in this case, three _
refer to different variables. An initial upgrade to this lambda code could exist to name the variables:
>>>
>>> ( lambda some_list : list ( map ( lambda n : n // ii , some_list)))([1,2,3,iv,5,six,vii,8,9,10]) [0, one, 1, 2, 2, three, iii, iv, 4, 5]
Absolutely, information technology'south still difficult to read. By still taking reward of a lambda
, a regular function would go a long way to render this code more readable, spreading the logic over a few lines and function calls:
>>>
>>> def div_items ( some_list ): div_by_two = lambda n: n // 2 render map(div_by_two, some_list) >>> list ( div_items ([ 1 , 2 , iii , four , five , 6 , 7 , 8 , 9 , 10 ]))) [0, 1, ane, 2, 2, three, 3, 4, 4, v]
This is still not optimal but shows you a possible path to make lawmaking, and Python lambda functions in particular, more than readable. In Alternatives to Lambdas, y'all'll learn to supersede map()
and lambda
with list comprehensions or generator expressions. This volition drastically better the readability of the code.
Python Classes
You can but should not write form methods as Python lambda functions. The following example is perfectly legal Python code but exhibits unconventional Python code relying on lambda
. For example, instead of implementing __str__
every bit a regular function, information technology uses a lambda
. Similarly, brand
and year
are properties likewise implemented with lambda functions, instead of regular functions or decorators:
course Motorcar : """Car with methods as lambda functions.""" def __init__ ( self , make , twelvemonth ): self . brand = brand self . yr = year brand = property ( lambda self : getattr ( self , '_brand' ), lambda self , value : setattr ( self , '_brand' , value )) year = holding ( lambda self : getattr ( self , '_year' ), lambda self , value : setattr ( self , '_year' , value )) __str__ = lambda self : f ' { self . brand } { cocky . year } ' # i: error E731 honk = lambda self : print ( 'Honk!' ) # two: error E731
Running a tool like flake8
, a mode guide enforcement tool, will display the post-obit errors for __str__
and honk
:
E731 do not assign a lambda expression, employ a def
Although flake8
doesn't point out an effect for the usage of the Python lambda functions in the properties, they are difficult to read and prone to error because of the usage of multiple strings similar '_brand'
and '_year'
.
Proper implementation of __str__
would be expected to be as follows:
def __str__ ( cocky ): return f ' { cocky . brand } { self . yr } '
brand
would be written as follows:
@property def make ( self ): return self . _brand @brand . setter def brand ( cocky , value ): self . _brand = value
Every bit a general rule, in the context of code written in Python, adopt regular functions over lambda expressions. Nonetheless, at that place are cases that benefit from lambda syntax, every bit yous will see in the side by side section.
Appropriate Uses of Lambda Expressions
Lambdas in Python tend to be the subject of controversies. Some of the arguments against lambdas in Python are:
- Issues with readability
- The imposition of a functional way of thinking
- Heavy syntax with the
lambda
keyword
Despite the heated debates questioning the mere existence of this feature in Python, lambda functions have backdrop that sometimes provide value to the Python linguistic communication and to developers.
The following examples illustrate scenarios where the use of lambda functions is not only suitable merely encouraged in Python code.
Classic Functional Constructs
Lambda functions are regularly used with the built-in functions map()
and filter()
, too as functools.reduce()
, exposed in the module functools
. The following three examples are respective illustrations of using those functions with lambda expressions as companions:
>>>
>>> list ( map ( lambda 10 : x . upper (), [ 'cat' , 'dog' , 'moo-cow' ])) ['Cat', 'DOG', 'COW'] >>> list ( filter ( lambda ten : 'o' in 10 , [ 'cat' , 'domestic dog' , 'moo-cow' ])) ['dog', 'cow'] >>> from functools import reduce >>> reduce ( lambda acc , 10 : f ' { acc } | { x } ' , [ 'cat' , 'canis familiaris' , 'moo-cow' ]) 'cat | canis familiaris | cow'
You may have to read code resembling the examples to a higher place, admitting with more than relevant data. For that reason, it'south of import to recognize those constructs. Nevertheless, those constructs have equivalent alternatives that are considered more than Pythonic. In Alternatives to Lambdas, yous'll acquire how to convert higher-guild functions and their accompanying lambdas into other more than idiomatic forms.
Central Functions
Central functions in Python are higher-order functions that take a parameter central
as a named argument. key
receives a function that can be a lambda
. This function directly influences the algorithm driven by the key function itself. Here are some key functions:
-
sort()
: list method -
sorted()
,min()
,max()
: built-in functions -
nlargest()
andnsmallest()
: in the Heap queue algorithm moduleheapq
Imagine that y'all want to sort a list of IDs represented as strings. Each ID is the concatenation of the cord id
and a number. Sorting this list with the built-in part sorted()
, past default, uses a lexicographic order as the elements in the list are strings.
To influence the sorting execution, you can assign a lambda to the named argument key
, such that the sorting will use the number associated with the ID:
>>>
>>> ids = [ 'id1' , 'id2' , 'id30' , 'id3' , 'id22' , 'id100' ] >>> print ( sorted ( ids )) # Lexicographic sort ['id1', 'id100', 'id2', 'id22', 'id3', 'id30'] >>> sorted_ids = sorted ( ids , cardinal = lambda x : int ( x [ 2 :])) # Integer sort >>> print ( sorted_ids ) ['id1', 'id2', 'id3', 'id22', 'id30', 'id100']
UI Frameworks
UI frameworks like Tkinter, wxPython, or .NET Windows Forms with IronPython take advantage of lambda functions for mapping actions in response to UI events.
The naive Tkinter programme below demonstrates the usage of a lambda
assigned to the command of the Opposite push:
import tkinter as tk import sys window = tk . Tk () window . grid_columnconfigure ( 0 , weight = ane ) window . title ( "Lambda" ) window . geometry ( "300x100" ) label = tk . Label ( window , text = "Lambda Calculus" ) label . grid ( column = 0 , row = 0 ) button = tk . Push button ( window , text = "Reverse" , command = lambda : label . configure ( text = characterization . cget ( "text" )[:: - 1 ]), ) button . grid ( cavalcade = 0 , row = one ) window . mainloop ()
Clicking the push button Opposite fires an event that triggers the lambda function, irresolute the characterization from Lambda Calculus to suluclaC adbmaL*:
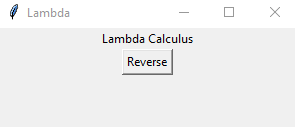
Both wxPython and IronPython on the .NET platform share a similar approach for handling events. Note that lambda
is one way to handle firing events, simply a function may be used for the same purpose. It ends up being self-independent and less verbose to utilize a lambda
when the corporeality of code needed is very curt.
To explore wxPython, check out How to Build a Python GUI Awarding With wxPython.
Python Interpreter
When you're playing with Python code in the interactive interpreter, Python lambda functions are often a blessing. Information technology'due south easy to craft a quick one-liner office to explore some snippets of code that will never meet the lite of day exterior of the interpreter. The lambdas written in the interpreter, for the sake of speedy discovery, are like fleck paper that you can throw away later use.
timeit
In the same spirit as the experimentation in the Python interpreter, the module timeit
provides functions to time minor code fragments. timeit.timeit()
in item can exist called directly, passing some Python lawmaking in a string. Here's an example:
>>>
>>> from timeit import timeit >>> timeit ( "factorial(999)" , "from math import factorial" , number = 10 ) 0.0013087529951008037
When the statement is passed as a string, timeit()
needs the full context. In the example above, this is provided by the second argument that sets up the environment needed by the main function to be timed. Not doing so would enhance a NameError
exception.
Another approach is to use a lambda
:
>>>
>>> from math import factorial >>> timeit ( lambda : factorial ( 999 ), number = 10 ) 0.0012704220062005334
This solution is cleaner, more readable, and quicker to type in the interpreter. Although the execution time was slightly less for the lambda
version, executing the functions once more may show a slight advantage for the string
version. The execution time of the setup
is excluded from the overall execution time and shouldn't take any impact on the result.
Monkey Patching
For testing, information technology's sometimes necessary to rely on repeatable results, fifty-fifty if during the normal execution of a given software, the respective results are expected to differ, or fifty-fifty exist totally random.
Let's say yous want to exam a function that, at runtime, handles random values. But, during the testing execution, yous demand to affirm against predictable values in a repeatable mode. The following example shows how, with a lambda
function, monkey patching can help you lot:
from contextlib import contextmanager import secrets def gen_token (): """Generate a random token.""" return f 'TOKEN_ { secrets . token_hex ( eight ) } ' @contextmanager def mock_token (): """Context manager to monkey patch the secrets.token_hex office during testing. """ default_token_hex = secrets . token_hex secrets . token_hex = lambda _ : 'feedfacecafebeef' yield secrets . token_hex = default_token_hex def test_gen_key (): """Test the random token.""" with mock_token (): assert gen_token () == f "TOKEN_ { 'feedfacecafebeef' } " test_gen_key ()
A context manager helps with insulating the operation of monkey patching a office from the standard library (secrets
, in this example). The lambda function assigned to secrets.token_hex()
substitutes the default behavior by returning a static value.
This allows testing any function depending on token_hex()
in a predictable way. Prior to exiting from the context manager, the default behavior of token_hex()
is reestablished to eliminate any unexpected side effects that would affect other areas of the testing that may depend on the default behavior of token_hex()
.
Unit examination frameworks like unittest
and pytest
take this concept to a higher level of sophistication.
With pytest
, even so using a lambda
function, the same example becomes more elegant and concise :
import secrets def gen_token (): return f 'TOKEN_ { secrets . token_hex ( 8 ) } ' def test_gen_key ( monkeypatch ): monkeypatch . setattr ( 'secrets.token_hex' , lambda _ : 'feedfacecafebeef' ) assert gen_token () == f "TOKEN_ { 'feedfacecafebeef' } "
With the pytest monkeypatch
fixture, secrets.token_hex()
is overwritten with a lambda that volition render a deterministic value, feedfacecafebeef
, allowing to validate the examination. The pytest monkeypatch
fixture allows you to control the scope of the override. In the example above, invoking secrets.token_hex()
in subsequent tests, without using monkey patching, would execute the normal implementation of this office.
Executing the pytest
test gives the following result:
$ pytest test_token.py -v ============================= test session starts ============================== platform linux -- Python iii.seven.2, pytest-four.3.0, py-1.viii.0, pluggy-0.ix.0 cachedir: .pytest_cache rootdir: /home/andre/AB/tools/bpython, inifile: collected 1 detail test_token.py::test_gen_key PASSED [100%] =========================== one passed in 0.01 seconds ===========================
The test passes every bit we validated that the gen_token()
was exercised, and the results were the expected ones in the context of the test.
Alternatives to Lambdas
While there are groovy reasons to use lambda
, in that location are instances where its employ is frowned upon. So what are the alternatives?
Higher-lodge functions similar map()
, filter()
, and functools.reduce()
can exist converted to more elegant forms with slight twists of creativity, in particular with list comprehensions or generator expressions.
To acquire more about list comprehensions, check out When to Utilize a Listing Comprehension in Python. To larn more than nigh generator expressions, check out How to Utilise Generators and yield in Python.
Map
The built-in function map()
takes a function as a first argument and applies it to each of the elements of its second argument, an iterable. Examples of iterables are strings, lists, and tuples. For more information on iterables and iterators, bank check out Iterables and Iterators.
map()
returns an iterator corresponding to the transformed drove. Every bit an example, if yous wanted to transform a listing of strings to a new list with each string capitalized, you could use map()
, as follows:
>>>
>>> listing ( map ( lambda x : x . capitalize (), [ 'true cat' , 'dog' , 'moo-cow' ])) ['Cat', 'Dog', 'Cow']
You need to invoke list()
to convert the iterator returned by map()
into an expanded list that can be displayed in the Python vanquish interpreter.
Using a list comprehension eliminates the need for defining and invoking the lambda office:
>>>
>>> [ ten . capitalize () for x in [ 'cat' , 'domestic dog' , 'cow' ]] ['Cat', 'Dog', 'Cow']
Filter
The congenital-in role filter()
, another archetype functional construct, can be converted into a list comprehension. Information technology takes a predicate as a first argument and an iterable equally a second argument. It builds an iterator containing all the elements of the initial collection that satisfies the predicate function. Here'southward an case that filters all the even numbers in a given list of integers:
>>>
>>> even = lambda x : x % two == 0 >>> listing ( filter ( fifty-fifty , range ( eleven ))) [0, 2, 4, 6, 8, 10]
Note that filter()
returns an iterator, hence the need to invoke the built-in type list
that constructs a list given an iterator.
The implementation leveraging the listing comprehension construct gives the post-obit:
>>>
>>> [ ten for x in range ( 11 ) if x % 2 == 0 ] [0, two, 4, 6, viii, 10]
Reduce
Since Python iii, reduce()
has gone from a built-in function to a functools
module function. Every bit map()
and filter()
, its start ii arguments are respectively a function and an iterable. It may also accept an initializer as a 3rd argument that is used as the initial value of the resulting accumulator. For each element of the iterable, reduce()
applies the function and accumulates the result that is returned when the iterable is exhausted.
To employ reduce()
to a list of pairs and calculate the sum of the offset item of each pair, you could write this:
>>>
>>> import functools >>> pairs = [( ane , 'a' ), ( 2 , 'b' ), ( 3 , 'c' )] >>> functools . reduce ( lambda acc , pair : acc + pair [ 0 ], pairs , 0 ) six
A more idiomatic approach using a generator expression, equally an statement to sum()
in the example, is the post-obit:
>>>
>>> pairs = [( 1 , 'a' ), ( 2 , 'b' ), ( 3 , 'c' )] >>> sum ( x [ 0 ] for ten in pairs ) 6
A slightly different and possibly cleaner solution removes the need to explicitly access the first element of the pair and instead employ unpacking:
>>>
>>> pairs = [( 1 , 'a' ), ( 2 , 'b' ), ( 3 , 'c' )] >>> sum ( x for x , _ in pairs ) half-dozen
The use of underscore (_
) is a Python convention indicating that you lot tin ignore the second value of the pair.
sum()
takes a unique argument, so the generator expression does non need to be in parentheses.
Are Lambdas Pythonic or Not?
PEP 8, which is the fashion guide for Python code, reads:
Always use a def statement instead of an assignment statement that binds a lambda expression directly to an identifier. (Source)
This strongly discourages using lambda bound to an identifier, mainly where functions should be used and have more than benefits. PEP 8 does not mention other usages of lambda
. Equally you take seen in the previous sections, lambda functions may certainly have good uses, although they are express.
A possible style to answer the question is that lambda functions are perfectly Pythonic if in that location is nothing more Pythonic available. I'm staying away from defining what "Pythonic" means, leaving yous with the definition that best suits your mindset, likewise as your personal or your squad's coding style.
Beyond the narrow scope of Python lambda
, How to Write Beautiful Python Code With PEP viii is a great resource that you may want to cheque out regarding lawmaking manner in Python.
Conclusion
You now know how to use Python lambda
functions and tin:
- Write Python lambdas and utilise anonymous functions
- Choose wisely betwixt lambdas or normal Python functions
- Avoid excessive apply of lambdas
- Use lambdas with college-order functions or Python key functions
If you have a penchant for mathematics, you may have some fun exploring the fascinating world of lambda calculus.
Python lambdas are like salt. A pinch in your spam, ham, and eggs will enhance the flavors, but as well much will spoil the dish.
Lookout Now This tutorial has a related video course created by the Real Python squad. Picket it together with the written tutorial to deepen your agreement: How to Apply Python Lambda Functions
dobsonalienighted.blogspot.com
Source: https://realpython.com/python-lambda/
0 Response to "How to Call a Function Again if False Python"
Post a Comment